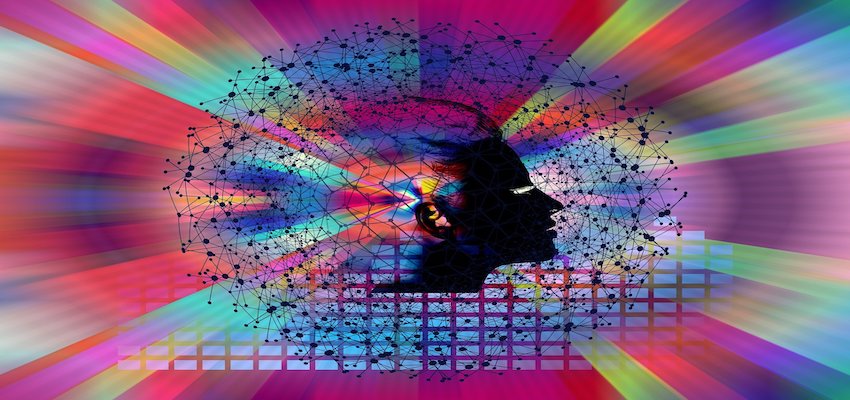
Bootstrap Website running as nodejs app
First I design the HTML site. The website is a simple Bootstrap-based site. This HTML part has the following tree structure.
.
├── images
│ ├── 2022-05-28-1-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-2-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-3-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-4-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-07-09-Gianni-Motta-1.jpeg
│ ├── 2022-07-09-Gianni-Motta-2.jpeg
│ └── 2022-07-09-Gianni-Motta-3.jpeg
└── index.html
The index.html contain the HTML and include a navbar, a hero section, and a footer. The index.html looks like the following.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Funtrails - Nodejs Demo</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/css/bootstrap.min.css" rel="stylesheet">
</head>
<body>
<!-- Navbar -->
<nav class="navbar navbar-expand-lg navbar-dark bg-dark">
<div class="container">
<a class="navbar-brand" href="#">Funtrails - Demo</a>
<button class="navbar-toggler" type="button" data-bs-toggle="collapse" data-bs-target="#navbarNav">
<span class="navbar-toggler-icon"></span>
</button>
<div class="collapse navbar-collapse" id="navbarNav">
<ul class="navbar-nav ms-auto">
<li class="nav-item"><a class="nav-link" href="#paterlini">Paterlini</a></li>
<li class="nav-item"><a class="nav-link" href="#motta">Gianni Motta</a></li>
</ul>
</div>
</div>
</nav>
<!-- Hero Section -->
<header class="bg-primary text-white text-center py-5">
<div class="container">
<h1>Funtrails</h1>
<p class="lead">History of Bikes and Components</p>
</div>
</header>
<!-- Patrlini Bike Section -->
<section id="paterlini" class="py-5">
<div class="container">
<h2 class="text-center">Paterlini</h2>
<p class="text-center">Paterlini Road Steel Bike from 1982</p>
<div id="paterliniCarousel" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<img src="images/2022-05-28-1-Paterlini-Umbau-80s-Ansicht.jpeg" class="d-block w-100" alt="Mountain Bike 1">
</div>
<div class="carousel-item">
<img src="images/2022-05-28-4-Paterlini-Umbau-80s-Ansicht.jpeg" class="d-block w-100" alt="Mountain Bike 2">
</div>
<div class="carousel-item">
<img src="images/2022-05-28-3-Paterlini-Umbau-80s-Ansicht.jpeg" class="d-block w-100" alt="Mountain Bike 3">
</div>
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#paterliniCarousel" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#paterliniCarousel" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
</button>
</div>
</div>
</section>
<!-- Gianni Motta Bike Section -->
<section id="motta" class="py-5">
<div class="container">
<h2 class="text-center">Gianni Motta Personal 2001R</h2>
<p class="text-center">Gianni Motta Road Steel Bike from 1989</p>
<div id="mottaCarousel" class="carousel slide" data-bs-ride="carousel">
<div class="carousel-inner">
<div class="carousel-item active">
<img src="images/2022-07-09-Gianni-Motta-1.jpeg" class="d-block w-100" alt="Road Bike 1">
</div>
<div class="carousel-item">
<img src="images/2022-07-09-Gianni-Motta-2.jpeg" class="d-block w-100" alt="Road Bike 2">
</div>
<div class="carousel-item">
<img src="images/2022-07-09-Gianni-Motta-3.jpeg" class="d-block w-100" alt="Road Bike 3">
</div>
</div>
<button class="carousel-control-prev" type="button" data-bs-target="#mottaCarousel" data-bs-slide="prev">
<span class="carousel-control-prev-icon" aria-hidden="true"></span>
</button>
<button class="carousel-control-next" type="button" data-bs-target="#mottaCarousel" data-bs-slide="next">
<span class="carousel-control-next-icon" aria-hidden="true"></span>
</button>
</div>
</div>
</section>
<!-- Footer -->
<footer class="bg-dark text-white text-center py-3">
<p>© 2025 Funtrails. All rights reserved.</p>
</footer>
<script src="https://cdn.jsdelivr.net/npm/bootstrap@5.3.0/dist/js/bootstrap.bundle.min.js"></script>
</body>
</html>
This website is a one-page bicycle showcase with two sections: one for my Paterlini bike and one for my Gianni Motta bike, each containing a gallery and text. The image galleries in the Bike sections are Bootstrap carousels that allow users to slide through images.
Then I create a new file structure for my node app. Within the funtrails directory I create a views directory. This is the location where my HTML part will be located. Then I create a package.json file just under funtrails. I copy the images folder and the index.html file from the HTML part above into the views folder of my new structure. The new structure looks like this.
funtrails
├── package.json
└── views
├── images
│ ├── 2022-05-28-1-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-2-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-3-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-4-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-07-09-Gianni-Motta-1.jpeg
│ ├── 2022-07-09-Gianni-Motta-2.jpeg
│ └── 2022-07-09-Gianni-Motta-3.jpeg
└── index.html
The package.json file has the following code.
{
"name": "funrails-demo",
"version": "1.0.0",
"description": "nodejs funtrails website demo",
"author": "Patrick Rottlaender <p.rottlaendere@icloud.com>",
"license": "MIT",
"main": "app.js",
"keywords": [
"nodejs",
"bootstrap",
"express"
],
"dependencies": {
"express": "^4.16.4"
}
}
For my node app I use express framework in version 4.16.4 or above. The code of my app will be in the main file app.js. Then I run npm install to install all dependencies.
npm install
This create a new folder for my node modules and a package-lock.json file.
funtrails
├── node_modules
├── package.json
├── package-lock.json
└── views
├── images
│ ├── 2022-05-28-1-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-2-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-3-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-4-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-07-09-Gianni-Motta-1.jpeg
│ ├── 2022-07-09-Gianni-Motta-2.jpeg
│ └── 2022-07-09-Gianni-Motta-3.jpeg
└── index.html
I create the main app.js file with the following code.
//Load the express framework
const express = require('express');
//create express app
const app = express();
//create Router instance
const router = express.Router();
//path for static content
const path = __dirname + '/views/';
//set port
const port = process.env.PORT || 8080;
//create Middleware
router.use(function (req,res,next) {
console.log('/' + req.method);
next();
});
//create the route handlet for get
router.get('/', function(req,res){
res.sendFile(path + 'index.html');
});
//app will use the static path and the router
app.use(express.static(path));
app.use('/', router);
//app listens on localhost port 8080
app.listen(port, function () {
console.log('nodejs funtrails demo app listening on port 8080!')
})
Let’s go through this Node.js code line by line.
- require(‚express‘) loads the Express module. This allows us to use express functionalities in our application.
- Calls the express() function to create an Express application. The app object will be used to define routes, middleware, and configure the server.
- Creates a new router instance using express.Router(). The router allows grouping route handlers separately from the main app.
- dirname refers to the directory of the current JavaScript file. Concatenating ‚/views/‘ results in a full path to the views directory. This will be used to serve static HTML files and images.
- process.env.PORT checks if a port number is set in the environment variables. If not set, it defaults to 8080. This allows the application to use a dynamic port in cloud environments while using 8080 locally.
- This middleware logs the HTTP request method (e.g., GET, POST). req.method returns the request type. next() passes control to the next middleware or route handler. Example logs when requests are made: /GET, /POST
- Defines a route handler for GET / (the home page). Uses res.sendFile(path + ‚index.html‘) to send index.html from the views directory.
- express.static(path): Serves static files (CSS, JS, images) from the views directory.
- app.use(‚/‘, router): Registers the router for handling requests.
- Starts the server on the specified port. Logs a message indicating that the server is running.
The final file structure is as follows.
funtrails
├── app.js
├── node_modules
├── package.json
├── package-lock.json
└── views
├── images
│ ├── 2022-05-28-1-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-2-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-3-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-05-28-4-Paterlini-Umbau-80s-Ansicht.jpeg
│ ├── 2022-07-09-Gianni-Motta-1.jpeg
│ ├── 2022-07-09-Gianni-Motta-2.jpeg
│ └── 2022-07-09-Gianni-Motta-3.jpeg
└── index.html
Run the app with node app.js.
node app.js